Direct Deposit
Introduction
Users can make updates to their payroll account's direct deposit settings within your application with Pinwheel. After users connect their account, the settings can be updated with a new allocation or adjusted for an existing allocation to an account you specify.
Use Cases
Direct Deposit Switch
Direct Deposit Switch enables users to update their direct deposit settings directly in your native experience. In just a few clicks, users can change where they receive part or all of their paycheck. To take advantage of this flow, you will use our direct_deposit_switch
job.
There are two types of allocations users can choose from:
- Full Switch: Switches the "main" direct deposit account (also referred to as a "balance" or "remainder" account) to the account specified in the Link token.
- Partial Switch: Allows users to switch a fixed amount or percentage to the account specified in the Link token. Note: not all platforms support partial switches, in which case users will only be given the full switch option.
- Fixed amount: allows users to specify a specific dollar amount from each paycheck (e.g. $500).
- Percentage: allows users to allocate a proportion of each paycheck (e.g. 20%).
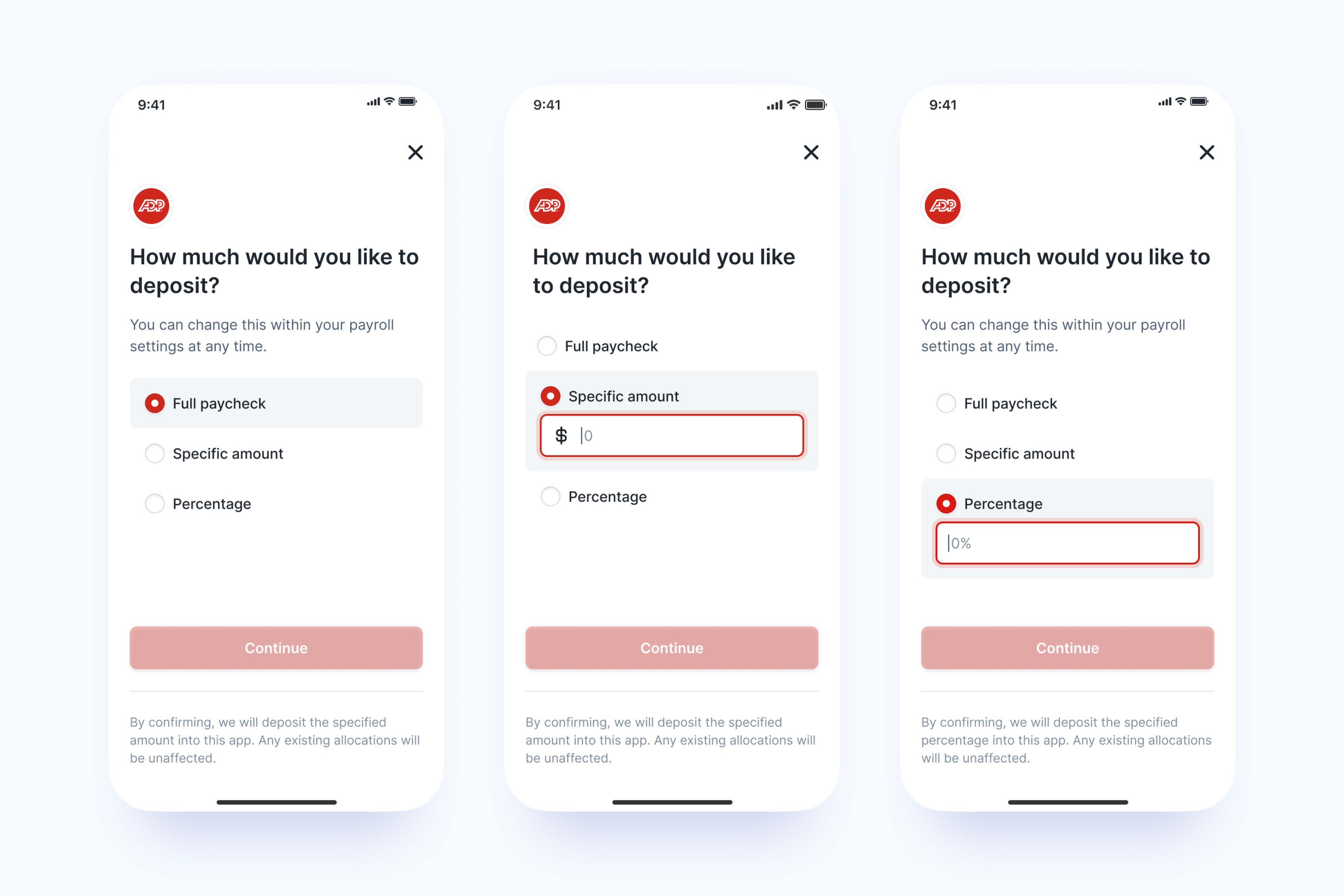
The partial switch options can be disabled when creating a Link Token using the optional disable_direct_deposit_splitting
parameter. This parameter is specific to direct_deposit_switch
job.
Paycheck-linked Loans
Paycheck-linked Loans allows your application to create a fixed amount direct deposit allocation to the account specified in the Link token. With Paycheck-linked Loans, borrowers can opt to have lenders collect payment directly from their paycheck. Here you will leverage our direct_deposit_switch
job and pass in the amount
parameter when creating a Link Token. When this parameter is passed in, users will not be able to edit the amount and is great for scenarios with a fixed payment amount.
The direct_deposit_switch
job will set up a recurring payment to the specified account. In order to stop a payment, have the user go through the Link flow again with amount: 0
.
Note
If an allocation already exists for the account specified in the Link token, the allocation will be updated to the new amount. For example if the existing allocation is $500, and you run a
direct_deposit_switch
job withamount
set to $400, the final allocation will be $400, not $900.
Direct Deposit Allocations
Direct Deposit Allocations allows your application to retrieve the existing allocations of a user's payroll account. The direct_deposit_allocations
job retrieves direct deposit configuration details including bank routing numbers, masked account numbers, amount, and priority of the existing allocations. See the full API reference here .
This job gives visibility into a user's account, but does not edit the direct deposit settings.
Implementation
Step 1: Subscribe to Webhook Events
The most reliable method to receive notifications of when direct deposit changes are successfully made is to subscribe to webhook events. Depending on the job, subscribe to either direct_deposit_switch.added
or direct_deposit_allocations.added
events:
POST /v1/webhooks
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"url": "https://your-domain.com/webhook_endpoint",
"status": "ACTIVE",
"enabled_events": [
"direct_deposit_switch.added"
]
}
The complete guide to webhooks can be found here.
Step 2: Create a Link token
Create a Link Token specifying the jobs that your application requires using the required_jobs
parameter. You can specify Income and Employment jobs in addition to direct_deposit_switch
. Please note that the search screen results will only return Platforms or Employers that support your required_jobs
.
For the direct_deposit_switch
job, you will also provide the direct deposit bank account details in the request: account_type
, routing_number
, and account_number
. The token creation examples below display the fields for each use case. To see all configuration options, please see the Link Token reference, and for examples of how to leverage the customization parameters see the Implementation Guide.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"account_type": "checking",
"routing_number": "07464755",
"account_number": "193464372203",
"required_jobs": [
"direct_deposit_switch"
]
}
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"account_type": "checking",
"routing_number": "07464755",
"account_number": "193464372203",
"amount": "50000",
"required_jobs": [
"direct_deposit_switch"
]
}
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"required_jobs": [
"direct_deposit_allocations"
]
}
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"account_type": "checking",
"routing_number": "07464755",
"account_number": "193464372203",
"required_jobs": [
"direct_deposit_switch"
],
"disable_direct_deposit_splitting": true
}
Note: The end_user_id
is your internal reference to the end user. See User Model for more information.
The response includes a short-lived token
that expires after 15 minutes and a unique id
. The token
will be used to initialize Link. Persist the id
in your database to query for job results later. More detail on storing identifiers can be found here.
{
"data": {
"id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"expires": "2021-01-09T02:52:26+00:00",
"mode": "production",
"token": "eyJ0eXAiOiJKV1QiLCJhbGci...cyldX8fILelb6A0XKmdWsXZHMH4W1o"
}
}
Step 3: Initialize Link
Using the Link token that was created, open the Link modal in your client application. In addition to passing in the token, you can optionally pass in several callback handlers.
<!DOCTYPE html>
<html>
<head>
<script src="https://cdn.getpinwheel.com/pinwheel-v2.3.js"></script>
<script>
Pinwheel.open({
linkToken: "INSERT LINK TOKEN",
onSuccess: (result) => {
console.log("Job succeeded!");
},
});
</script>
</head>
<body></body>
</html>
The onSuccess
callback handler is executed on job success and contains metadata about the direct deposit job.
Step 4: Responding to Webhook Events
After a user logs into their payroll account, an account.added
webhook event is published. Using the link_token_id
you persisted earlier, you can associate the account with the user who logged in with Link.
{
"event": "account.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"end_user_id": "my_user_12345",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"platform_id": "fce3eee0-285b-496f-9b36-30e976194736",
"created_at": "2021-01-12T02:36:01.287148+00:00",
"connected": true
}
}
Depending on the job executed, a direct_deposit_switch.added
or direct_deposit_allocations.added
webhook event is published. For example:
{
"event": "direct_deposit_switch.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"end_user_id": "my_user_12345",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"name": "direct_deposit_switch",
"timestamp": "2021-01-12T02:36:01.287148+00:00",
"outcome": "success",
"params": {
"amount": null
}
}
}
{
"event": "direct_deposit_allocations.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "449e7a5c-69d3-4b8a-aaaf-5c9b713ebc65",
"end_user_id": "my_user_12345",
"link_token_id": "4787acbc-11cf-4db3-998c-5ea7c4feebcd",
"name": "direct_deposit_allocations",
"timestamp": "2021-01-12T02:36:01.287148+00:00",
"outcome": "success"
}
}
Step 5: Query for Job Results
If your application never subscribed to webhook events, or your application server failed to handle the direct_deposit_switch.added
or direct_deposit_allocations.added
event, you can query the Jobs endpoint with the ID of the Link token used to initialize Link to fetch the results.
Request
GET /v1/jobs?link_token_id=97f420ff-5d0a-46ee-9cfc-6f17d5d31256
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
Response
{
"meta": {
"count": 1
},
"data": [
{
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"name": "direct_deposit_switch",
"timestamp": "2021-01-12T02:36:01.287148+00:00",
"outcome": "success",
"params": {
"amount": 50000
}
}
]
}
On Demand Updates
There are cases where you may want to take different actions on a payroll account. We recognize that asking the user to login multiple times introduces friction, so we've added the ability to re-access the payroll account without needing the user to re-enter credentials.
If the user has multi-factor authentication enabled on their account, user action may be required to complete the login step.
Sample use cases
- To increase share of wallet, you can incentivize users to increase their direct deposit allocation after an initial direct deposit switch. Users may initially allocate a small amount into the account, but with the right incentives, they may be willing to increase that amount.
- To help with underwriting decisions, you can leverage income and employment data before asking the user to complete a direct deposit switch.
- To reduce fraud, you can match the identity of the payroll account owner against your customer prior to allowing a direct deposit switch.
Implementation
Enabling this functionality is similar to the way standard Link tokens are created today. When creating the Link token, pass in the account_id
parameter from a prior account.added webhook event or from the login
client side event in Link. The account_id
can be preserved and passed in any time you have it for a user.
Taking the 3rd example above where you match the identity of the payroll account owner against that of your user, you would first create the Link token with identity
as the required job. Once your customer logs in and the job completes successfully, you will receive both the accounts.added
and identity.added
webhooks.
{
"event": "account.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"account_id": "449e7a5c-69d3-4b8a-aaaf-5c9b713ebc65",
"end_user_id": "my_user_12345",
"link_token_id": "4787acbc-11cf-4db3-998c-5ea7c4feebcd",
"platform_id": "fce3eee0-285b-496f-9b36-30e976194736",
"created_at": "2021-01-12T02:36:01.287148+00:00",
"connected": true
}
}
{
"event": "identity.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload":{
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "449e7a5c-69d3-4b8a-aaaf-5c9b713ebc65",
"end_user_id": "my_user_12345",
"link_token_id": "4787acbc-11cf-4db3-998c-5ea7c4feebcd",
"name": "identity",
"timestamp": "2021-01-12T02:36:01.287148+00:00",
"outcome": "success"
}
}
You can then query for full_name
, date_of_birth
, and last_four_ssn
in addition to other fields to determine if the payroll account owner is the same person as your user. For more info, please see the Income & Employment guide here.
Once you have successfully completed the verification, you can create another Link token to initiate the direct deposit switch using the same account_id
. In doing so, we will attempt to bypass the need for the user to re-enter credentials. Note that there are some cases, eg. where MFA is enabled, where the user will have to take an action to complete login.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
X-API-SECRET: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"account_type": "checking",
"routing_number": "07464755",
"account_number": "193464372203",
"required_jobs": [
"direct_deposit_switch"
],
"account_id": "449e7a5c-69d3-4b8a-aaaf-5c9b713ebc65"
}
Note: it is not necessary to pass end_user_id
when creating Link tokens for On Demand Updates. Webhooks returned from On Demand Updates will contain the end_user_id
you specified when the user first connected to their payroll account.
Please contact [email protected] for access to our Developer Dashboard.
Updated about 2 years ago