Implement Direct Deposit Switch
Direct Deposit Switch enables users to update their direct deposit settings directly in your native experience. In just a few clicks, users can change where they receive part or all of their paycheck. To take advantage of this flow, you can subscribe to webhook events, create a Link token with the direct_deposit_switch
job, and launch it in Link.
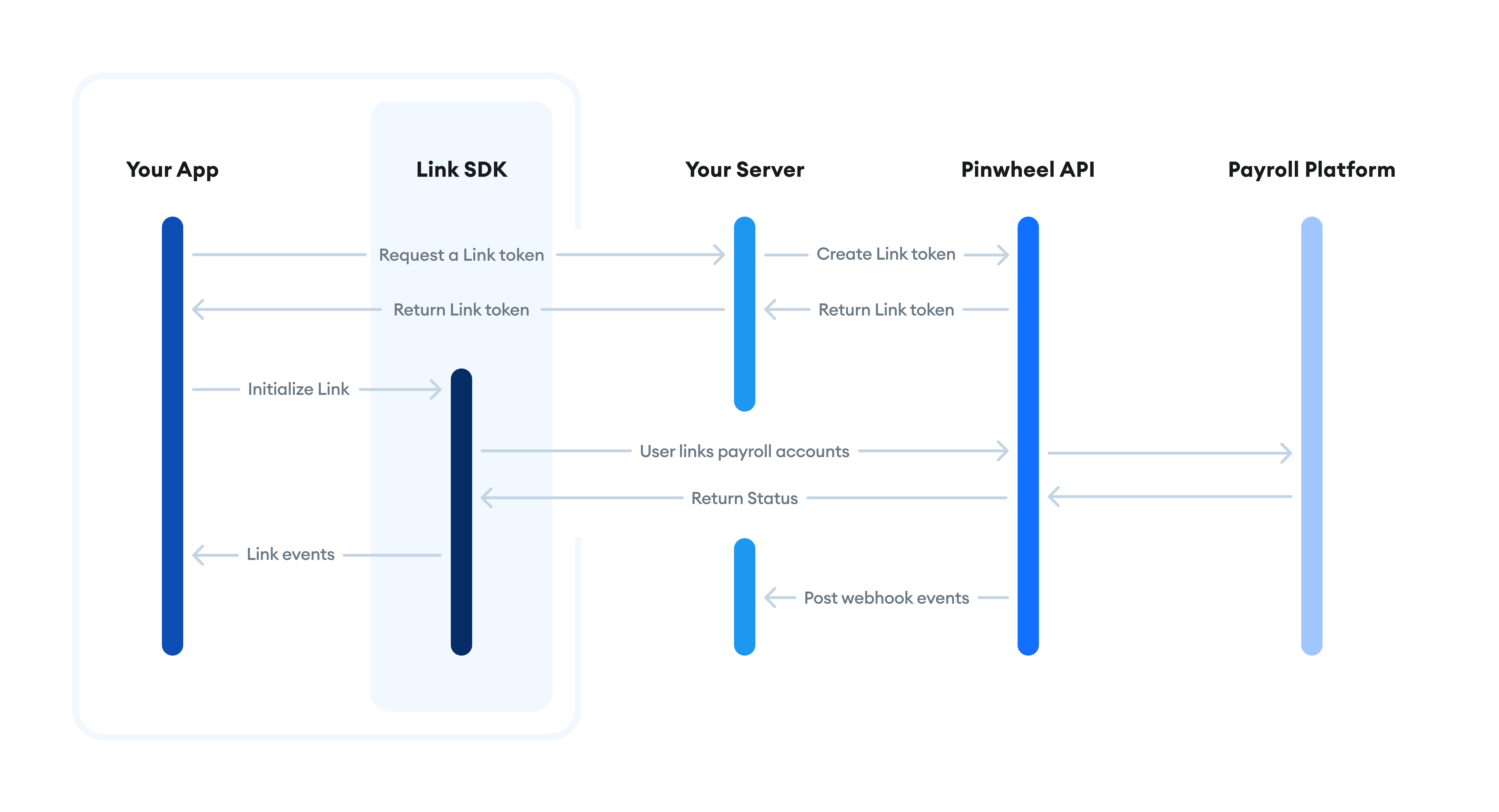
Step 1: Subscribe to Webhook Events
Subscribe to the following webhook events when changes to the user's direct deposit settings are made:
direct_deposit_switch.added
: Notifies you when information about the direct deposit switch is available.direct_deposit_allocations.added
: Notifies you when information about direct deposit allocations is available.
You can subscribe to either webhooks or both. Register a webhook endpoint using Pinwheel's Create Webhook, as the following example illustrates.
POST /v1/webhooks
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"url": "https://your-domain.com/webhook_endpoint",
"status": "ACTIVE",
"enabled_events": [
"direct_deposit_switch.added"
]
}
Step 2: Create a Link token
Start by creating a Link Token with the jobs that your application requires specified within the required_jobs
parameter. You can also combine Income and employment jobs to pull down payroll data within the same session.
Pinwheel search experience filters out results based on the
required_jobs
, i.e., Pinwheel only returns Platforms and Employers that meet your requirements.
Direct Deposit Switch
For direct_deposit_switch
provide details for the target bank account that you want the user to switch their direct deposit to. Each one needs to include type
, account_number
, and routing_number
.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"allocation": {
"targets": [
{
"type": "checking",
"routing_number": "07464755",
"account_number": "193464372203"
}
]
},
"required_jobs": [
"direct_deposit_switch"
]
}
You can pass in multiple accounts per Link token, and the user can decide which one to switch to. Pass name
parameter when utilizing multiple accounts. This field is displayed within the Pinwheel Link user interface and returned in the direct_deposit_switch.added
webhook so that you can determine the selected account.
The token creation examples below display the request fields for each use case.
Paycheck-linked loans
Leverage direct_deposit_switch
job and pass in the amount
parameter when creating a Link Token. When this parameter is passed in, users cannot edit the amount, which is great for scenarios with a fixed payment amount.
The direct_deposit_switch
job sets up a recurring payment to the specified account. To stop a payment, have the user go through the Link flow again with amount: 0
.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"allocation": {
"type": "amount",
"value": "50000",
"targets": [
{
"type": "checking",
"routing_number": "07464755",
"account_number": "193464372203"
}
]
},
"required_jobs": [
"direct_deposit_switch"
]
}
Note
If an allocation already exists for the account specified in the Link token, the allocation will be updated to the new amount. For example if the existing allocation is $500, and you run a
direct_deposit_switch
job withamount
set to $400, the final allocation will be $400, not $900.
Direct Deposit Allocations
Use the direct_deposit_allocations
job to retrieve direct deposit configuration details, including bank routing numbers, masked account numbers, amount, and priority of the existing allocations. See the full API reference here.
This job gives visibility into a user's account but does not edit the direct deposit settings. Use this to understand how the user distributes their paycheck and what your share of the wallet is.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"required_jobs": [
"direct_deposit_allocations"
]
}
Disable Partial Switches
Use the optional disable_direct_deposit_splitting
parameters to disable the partial switch options when creating a Link Token. This parameter is specific to thedirect_deposit_switch
job.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"allocation": {
"targets": [
{
"type": "checking",
"routing_number": "07464755",
"account_number": "193464372203"
}
]
},
"required_jobs": [
"direct_deposit_switch"
],
"disable_direct_deposit_splitting": true
}
To see all configuration options, see Create Link Token reference. For examples of leveraging the customization parameters, see Platform Overview.
The
end_user_id
is your internal reference to the user and is required in all Link tokens without anaccount_id
as of API version 2023-07-18. See User Model for more information.
The response to all these requests includes a short-lived token
that expires after one hour and a unique id
. The token
initializes Link. Persist the id
in your database to query for job results later. To view details on storing identifiers, see Identifiers. Treat the token
as opaque and do not use or rely on the information encoded in the token as it can change at any time.
{
"data": {
"id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"expires": "2021-01-09T02:52:26+00:00",
"mode": "production",
"token": "eyJ0eXAiOiJKV1QiLCJhbGci...cyldX8fILelb6A0XKmdWsXZHMH4W1o"
}
}
Step 3: Initialize Link
Use the Link token to open the Link modal in your client application. In addition to passing in the token, you can optionally pass several callback handlers.
<!DOCTYPE html>
<html>
<head>
<script src="https://cdn.getpinwheel.com/pinwheel-v2.3.js"></script>
<script>
Pinwheel.open({
linkToken: "INSERT LINK TOKEN",
onSuccess: (result) => {
console.log("Job succeeded!");
},
});
</script>
</head>
<body></body>
</html>
The onSuccess
callback handler is executed on job success and contains metadata about the direct deposit job.
Step 4: Respond to Webhook Events
After a user successfully logs into their payroll account, an account.added
webhook event is published. Using the link_token_id
, you can associate the account with the user who logged in with Link.
{
"event": "account.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"end_user_id": "my_user_12345",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"platform_id": "fce3eee0-285b-496f-9b36-30e976194736",
"created_at": "2021-01-12T02:36:01.287148+00:00",
"connected": true
}
}
Depending on the job executed, a direct_deposit_switch.added
or direct_deposit_allocations.added
webhook event is published. For example:
{
"event": "direct_deposit_switch.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"end_user_id": "my_user_12345",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"name": "direct_deposit_switch",
"timestamp": "2021-01-12T02:36:01.287148+00:00",
"outcome": "success",
"params": {
"action": "partial_switch",
"allocation": {
"type": "remainder",
"target": {
"account_type": "checking"
}
}
}
}
}
{
"event": "direct_deposit_allocations.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "449e7a5c-69d3-4b8a-aaaf-5c9b713ebc65",
"end_user_id": "my_user_12345",
"link_token_id": "4787acbc-11cf-4db3-998c-5ea7c4feebcd",
"name": "direct_deposit_allocations",
"timestamp": "2021-01-12T02:36:01.287148+00:00",
"outcome": "success"
}
}
If the automated direct deposit job is unsuccessful and the user elects to complete a direct deposit form, you can listen for the following Link events to determine their progress. For more information on Link events, please click Link Events.
name | description | payload |
---|---|---|
dd_form_begin | User has elected to begin the process of creating a direct deposit form. | {} |
dd_form_create | User has successfully created the form. | { url: string } |
dd_form_download | User has clicked "share" or "download" | {} |
Step 5: Query for Job Results
If you are not subscribed to webhook events, or there was an issue handling thedirect_deposit_switch.added
or direct_deposit_allocations.added
events, you can fetch job results by querying the Jobs endpoint with the link_token_id
of a specific user.
Request
GET /v1/jobs?link_token_id=97f420ff-5d0a-46ee-9cfc-6f17d5d31256
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
Response
{
"meta": {
"count": 1
},
"data": [
{
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"name": "direct_deposit_switch",
"timestamp": "2021-01-12T02:36:01.287148+00:00",
"outcome": "success",
"params": {
"action": "partial_switch",
"allocation": {
"type": "amount",
"value": 5400,
"target": {
"account_type": "checking"
}
}
}
}
]
}
Step 6: Perform an On Demand Update
When a user's account loses connection or you need the latest information for a user, asking the user to log in multiple times introduces friction. Pinwheel has added the ability to reaccess the payroll account without needing the user to re-enter credentials.
Enabling this functionality is similar to creating standard Link tokens. When creating the Link token, pass in the account_id
parameter from a prior account.added webhook event or from the login
client side event in Link. The account_id
can be preserved and passed in whenever you have it for a user.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
X-API-SECRET: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"allocation": {
"targets": [
{
"type": "checking",
"routing_number": "07464755",
"account_number": "193464372203"
}
]
},
"required_jobs": [
"direct_deposit_switch"
],
"account_id": "449e7a5c-69d3-4b8a-aaaf-5c9b713ebc65"
}
It is not necessary to pass end_user_id
when creating Link tokens for on-demand Updates, as the information persists from the initial connection.
Once you've created the Link token, follow Step 3 to initialize the Link. When the user is presented with the Pinwheel Link flow, they are directed to the payroll platform associated with their account, bypassing login if possible.
If multi-factor authentication is enabled on the user's account, user action is required to complete the login step.
Please contact [email protected] for access to our Dashboard.
Updated 6 months ago