Implement PreMatch
PreMatch Deposit Switch is a premium offering built on Pinwheel's partnerships with payroll platforms. It offers both a superior conversion and a streamlined user experience that does not rely on users knowing their payroll provider or credentials. Together, they directly help increase your deposits.
Please contact [email protected] for a demo or access to PreMatch Deposit Switch.
How it works
Pinwheel customers capture unique user identifiers such as social security number (SSN), telephone number, and zip code during user signup and Know Your Customer (KYC) checks. These data points also exist within payroll provider databases. PreMatch Deposit Switch works by collecting these data points and matching them across payroll platform databases to find eligible user accounts.
Once this matching has been completed, the user will be prompted to connect with any platforms they are eligible to set up a direct deposit switch. To enhance security and prevent fraud, Pinwheel will perform a multi-factor authentication (MFA) check with the user's contact methods to ensure they are the account owner. After this authentication is complete, the user completes the switch process.
User experience
The PreMatch user experience differs from Pinwheel's standard flow by immediately matching your user with eligible payroll platforms. After seeing the introductory screen, your user will see a loading animation the lookup process concludes, which usually takes a few seconds. If an active account is found with a payroll partner, the user will see a screen prompting them to continue with that platform. If the user is matched to multiple platforms, they will see all options.
After selecting a platform, the user will be prompted to select an MFA method and complete authentication. Finally, they will be dropped into the normal direct deposit switch screen to finish setting up their switch.
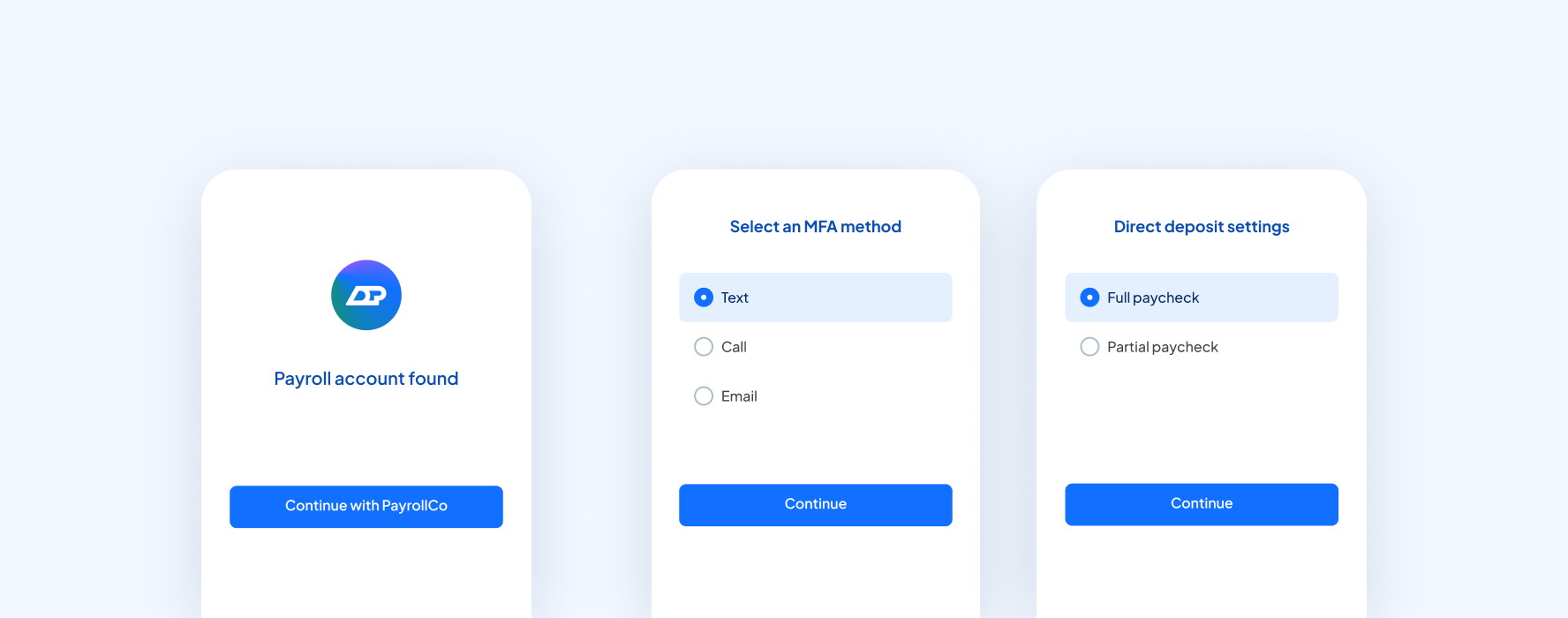
If no supported platforms are matched, the user will be routed to Pinwheel's search where they can lookup their employer or platform to complete a standard switch.
Implementation
Prerequisites
Link SDK version 3.0
In order to enable the credential-less platform matching feature, the Link SDK used in your application must be upgraded to version 3.0 or later.
The breaking change associated with this new SDK version is making the action
field of Link event input_allocation
optional. Additionally, when the action
key is not
present in webhook payload the allocation.value
and allocation.type
fields will also not be provided. Pinwheel does not receive this data from all payroll partners, so it can not be always provided in the client events.
API and Webhook Version v2023-11-22
Similar to the client events, the direct_deposit_switch.added
webhook will have its payload schema updated to reflect the absence of action
in some cases.
As a result of this breaking change, the API version used by your system’s backend must be upgraded to version 2023-11-22. To determine any other changes needed to be made to update to the latest version (if applicable), the list of breaking changes can be found here. In order receive valid webhook events of PreMatch switches, you must create a new webhook with version 2023-11-22. This can be done by using the Create Webhook endpoint. Old webhooks can be deleted using the Delete Webhook endpoint.
Step 1: Subscribe to Webhook Events
Subscribe to the following webhook events when changes to the user's direct deposit settings are made:
direct_deposit_switch.added
: Notifies you when information about the direct deposit switch is available.
POST /v1/webhooks
Host: api.getpinwheel.com
Content-Type: application/json
Pinwheel-Version: 2025-07-08
X-Api-Secret: YOUR-API-SECRET
{
"url": "https://your-domain.com/webhook_endpoint",
"status": "active",
"enabled_events": [
"direct_deposit_switch.added"
],
"version": "2023-11-22"
}
Step 2: Create a Link token for PreMatch switches
To enable Pinwheel Link to verify users’ eligibility for direct deposit switching, populate the end_user.platform_matching
field when creating a Link token with Create Link Token . If this field is populated, this data will automatically be used to look up the user's accounts with our payroll partners and find match platforms where the user has an active payroll account eligible for a direct deposit switch.
Key in end_user.platform_matching | Type | Description |
---|---|---|
social_security_number (required) | string | Full 9-digit social security number of the user. |
first_name (required) | string | The user's first name. |
last_name (required) | string | The user's last name. |
date_of_birth (required) | string | The user's date of birth in YYYY-MM-DD format. |
mobile_phone_number (required) | string | The users's 10-digit mobile phone number. Do not include country code. |
home_address_zip_code (required) | string | The users's 5-digit home zip code. |
email (strongly recommended) | string | The user's email. The email must have valid syntax with an @ and valid domain e.g. [email protected] . If provided email is invalid a 400 will be returned. For more details on validation see https://github.com/JoshData/python-email-validator. |
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
Pinwheel-Version: 2025-07-08
X-Api-Secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"end_user": {
"platform_matching": {
"social_security_number": "123456789",
"mobile_phone_number": "0123456789",
"home_address_zip_code": "12345",
"first_name": "John",
"last_name": "Smith",
"date_of_birth": "1999-12-31",
"email": "[email protected]"
}
},
"allocation": {
"targets": [
{
"type": "checking",
"routing_number": "07464755",
"account_number": "193464372203"
}
]
},
"solution": "Deposit Switch",
"features": [
"direct_deposit_switch"
]
}
As is the case for the normal Pinwheel user experience, Pinwheel will only check users’ platform matches if the associated platform supports all features
in the token and any other token restrictions. For example, including amount
or allocation.type.amount
and allocation.type.value
in the token will only search across partners who support pre-configured amounts.
It is also important to note that if a platform_id
or employer_id
is passed in the Link token, they will not receive the platform matching user flow and be routed directly to the chosen platform, negating many of the benefits of passing the end user data. As such, this feature should be used in conjunction with Pinwheel’s standard search.
The response to all these requests includes a short-lived token
that expires after one hour and a unique id
. The token
initializes Link. Persist the id
in your database to query for job results later. To view details on storing identifiers, see Identifiers. Treat the token
as opaque and do not use or rely on the information encoded in the token as it can change at any time.
{
"data": {
"id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"expires": "2023-04-09T02:52:26+00:00",
"mode": "production",
"token": "eyJ0eXAiOiJKV1QiLCJhbGci...cyldX8fILelb6A0XKmdWsXZHMH4W1o"
}
}
Step 3: Initialize Link
Use the Link token to open the Link modal in your client application. In addition to passing in the token, you can optionally pass several callback handlers.
<!DOCTYPE html>
<html>
<head>
<script src="https://cdn.getpinwheel.com/pinwheel-v2.3.js"></script>
<script>
Pinwheel.open({
linkToken: "INSERT LINK TOKEN",
onSuccess: (result) => {
console.log("Job succeeded!");
},
});
</script>
</head>
<body></body>
</html>
The onSuccess
callback handler is executed on job success and contains metadata about the direct deposit job.
Step 4: Respond to Webhook Events
After a user successfully logs into their payroll account, an account.added
webhook event is published. Using the end_user_id
, you can associate the account with the user who logged in with Link.
{
"event": "account.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"end_user_id": "my_user_12345",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"platform_id": "fce3eee0-285b-496f-9b36-30e976194736",
"created_at": "2023-01-12T02:36:01.287148+00:00",
"connected": true
}
}
When the direct_deposit_switch
job completes, a direct_deposit_switch.added
webhook event will be sent. For example:
{
"event": "direct_deposit_switch.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"end_user_id": "my_user_12345",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"name": "direct_deposit_switch",
"timestamp": "2023-04-12T02:36:01.287148+00:00",
"outcome": "success",
"params": {}
}
}
Unlike other switch types, the payload.params.action
field may be omitted when Pinwheel does not receive information about the action from our partners.
Step 5: Query for Job Results
If you are not subscribed to webhook events, or there was an issue handling thedirect_deposit_switch.added
event, you can fetch job results by querying the Jobs endpoint with the Link token id
used in the switch.
Request
GET /v1/jobs?link_token_id=97f420ff-5d0a-46ee-9cfc-6f17d5d31256
Host: api.getpinwheel.com
Content-Type: application/json
Pinwheel-Version: 2025-07-08
X-Api-Secret: YOUR-API-SECRET
Response
{
"meta": {
"count": 1
},
"data": [
{
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"name": "direct_deposit_switch",
"timestamp": "2023-01-12T02:36:01.287148+00:00",
"outcome": "success",
"params": {
"action": "partial_switch",
"allocation": {
"type": "amount",
"value": 5400,
"target": {
"account_type": "checking"
}
}
}
}
]
}
Step 6: Perform an On Demand Update
Enabling this functionality for the partner based product is similar to that of the standard direct deposit switch product. When creating the Link token, pass in the account_id
parameter from a prior account.added webhook event or from the login
client side event in Link. The account_id
can be preserved and passed in whenever you have it for a user. If the platform_name
contains the string (Partnership)
then the utilized platform was a partner platform.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
Pinwheel-Version: 2025-07-08
X-API-SECRET: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"allocation": {
"targets": [
{
"type": "checking",
"routing_number": "07464755",
"account_number": "193464372203"
}
]
},
"end_user": {
"platform_matching": {
"social_security_number": "123456789",
"mobile_phone_number": "0123456789",
"home_address_zip_code": "12345",
"first_name": "John",
"last_name": "Smith",
"date_of_birth": "1999-12-31",
"email": "[email protected]"
}
},
"solution": "Deposit Switch",
"features": [
"direct_deposit_switch"
],
"account_id": "449e7a5c-69d3-4b8a-aaaf-5c9b713ebc65"
}
It is not necessary to pass end_user_id
when creating Link tokens for on-demand Updates, as the information persists from the initial connection. The end_user.platform_matching
data will need to be provided again for On Demand Updates for partner based platforms due to automatic data deletion of user's PII from our system for their security.
Once you've created the Link token, follow Step 3 to initialize the Link. When the user is presented with the Pinwheel Link flow, they are directed to the payroll platform associated with their account, bypassing login if possible.
If multi-factor authentication is enabled on the user's account, user action is required to complete the login step.
Please contact [email protected] for access to our Dashboard.
Updated 3 days ago