iOS SDK
Installation
The Pinwheel iOS SDK is available via Swift Package Manager and CocoaPods. We recommend pinning to a specific version, thereby giving you explicit control over upgrades.
You can find the source code for the iOS SDK here.
Swift Package Manager
Xcode UI:
Note
Please use the latest available version listed here in the steps below.
From either the File menu in XCode, or the Swift Packages section of the project settings, use the XCode UI to add: https://github.com/underdog-tech/pinwheel-ios-sdk
as a package dependency, and set the exact version as the latest version retrieved above.
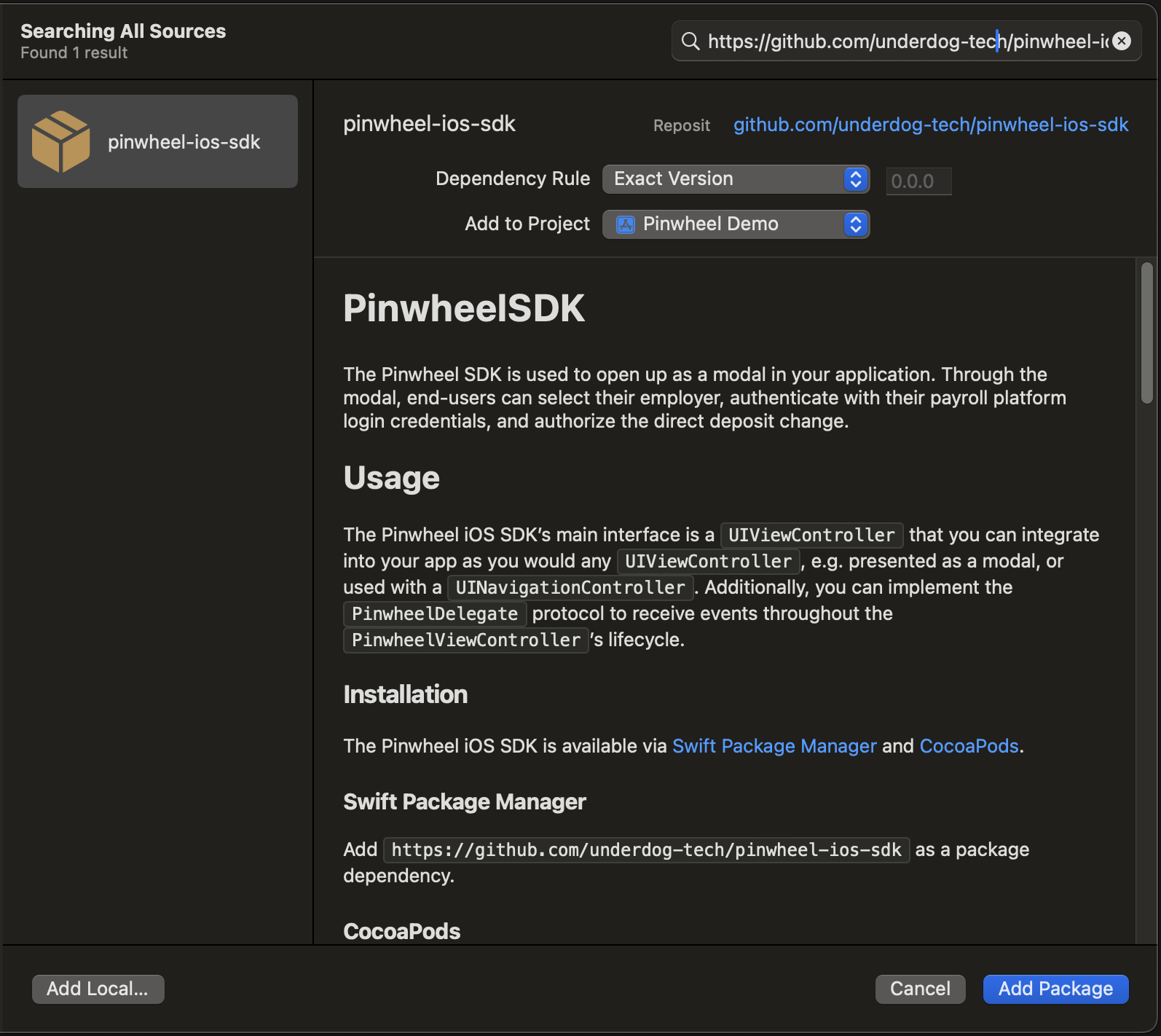
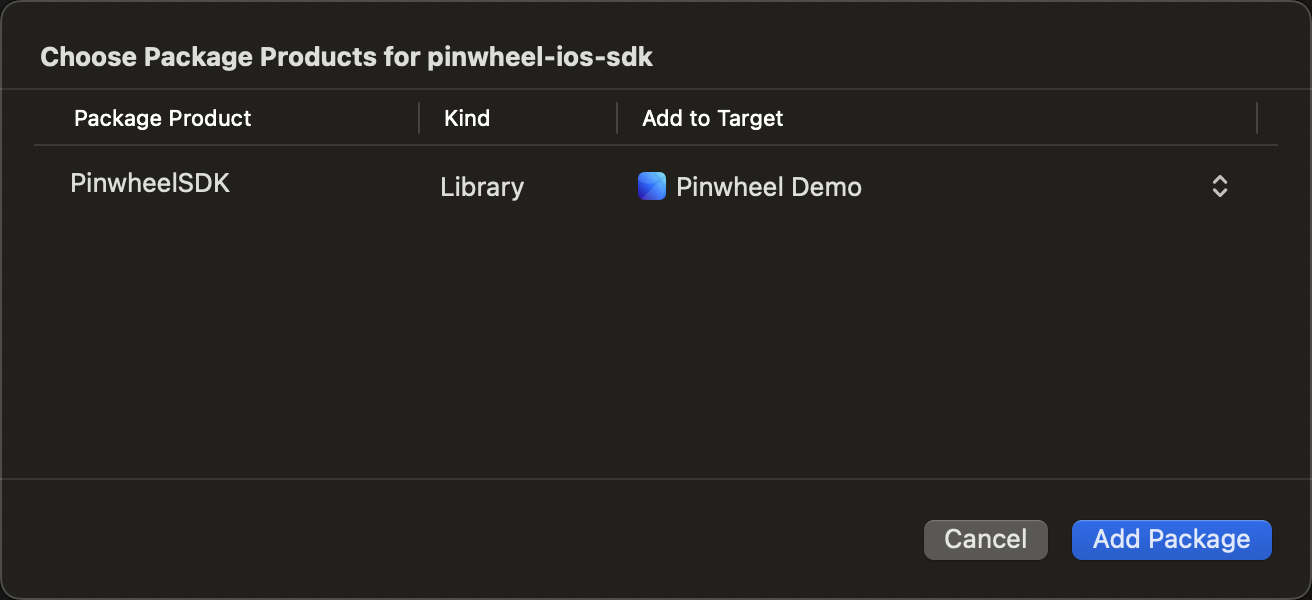
Package.swift
Add .package(url: "https://github.com/underdog-tech/pinwheel-ios-sdk", .exact("3.1.1"))
to your dependencies:
dependencies: [
.package(url: "https://github.com/underdog-tech/pinwheel-ios-sdk", .exact("3.1.1"))
],
CocoaPods
To install the SDK with CocoaPods, add PinwheelSDK
as one of your target dependencies in your Podfile:
use_frameworks!
target 'MyApp' do
pod 'PinwheelSDK', '3.1.1'
end
Please be sure to run pod update
and use pod install --repo-update
to ensure you have the most recent version of the SDK installed.
Configuration
Some platform integrations may require camera access for verification purposes. Ensure that the NSCameraUsageDescription
key is included in your Info.plist
:
<key>NSCameraUsageDescription</key>
<string>We need access to your camera for verification purposes.</string>
Usage
The iOS SDK's main interface is a UIViewController
that you can integrate into your app as you would any UIViewController
, e.g., presented as a modal, or used with a UINavigationController
. Additionally, you can implement the PinwheelDelegate
protocol to receive events throughout the PinwheelViewController
's lifecycle.
PinwheelViewController
The PinwheelViewController
is a UIViewController
that you can present as a modal.
import PinwheelSDK
let pinwheelVC = PinwheelViewController(token: linkToken, delegate: self)
self.present(pinwheelVC!, animated: true)
PinwheelDelegate
The PinwheelDelegate
protocol is set up such that every event goes through the onEvent(name: event:)
handler, and convenience callback methods are provided for the .exit
, .success
, .login
, and .error
events. Note that the onEvent(name: event:)
handler will still be called alongside the convenience methods.
You can see a full descriptions of props and optional callbacks here. Callback types can be found in Github.
Example Project
To run the example project, clone the repo, and run pod install
from the Example directory first. Then, add your API secret to the top of the LinkToken.swift
file. In your app, you should fetch the Link token from your server.
Maintenance
Pinwheel regularly releases improvements to this SDK. You should update to the latest version of the iOS SDK at least quarterly to ensure the best product performance.
Updated 3 months ago