Implement Verification Reports
Pinwheel Verify provides ongoing access to income and employment data directly from payroll platforms. You can access verification reports to verify customers' information, manage lending risk, improve the loan application process, and more.
Verification reports are associated with an end_user_id
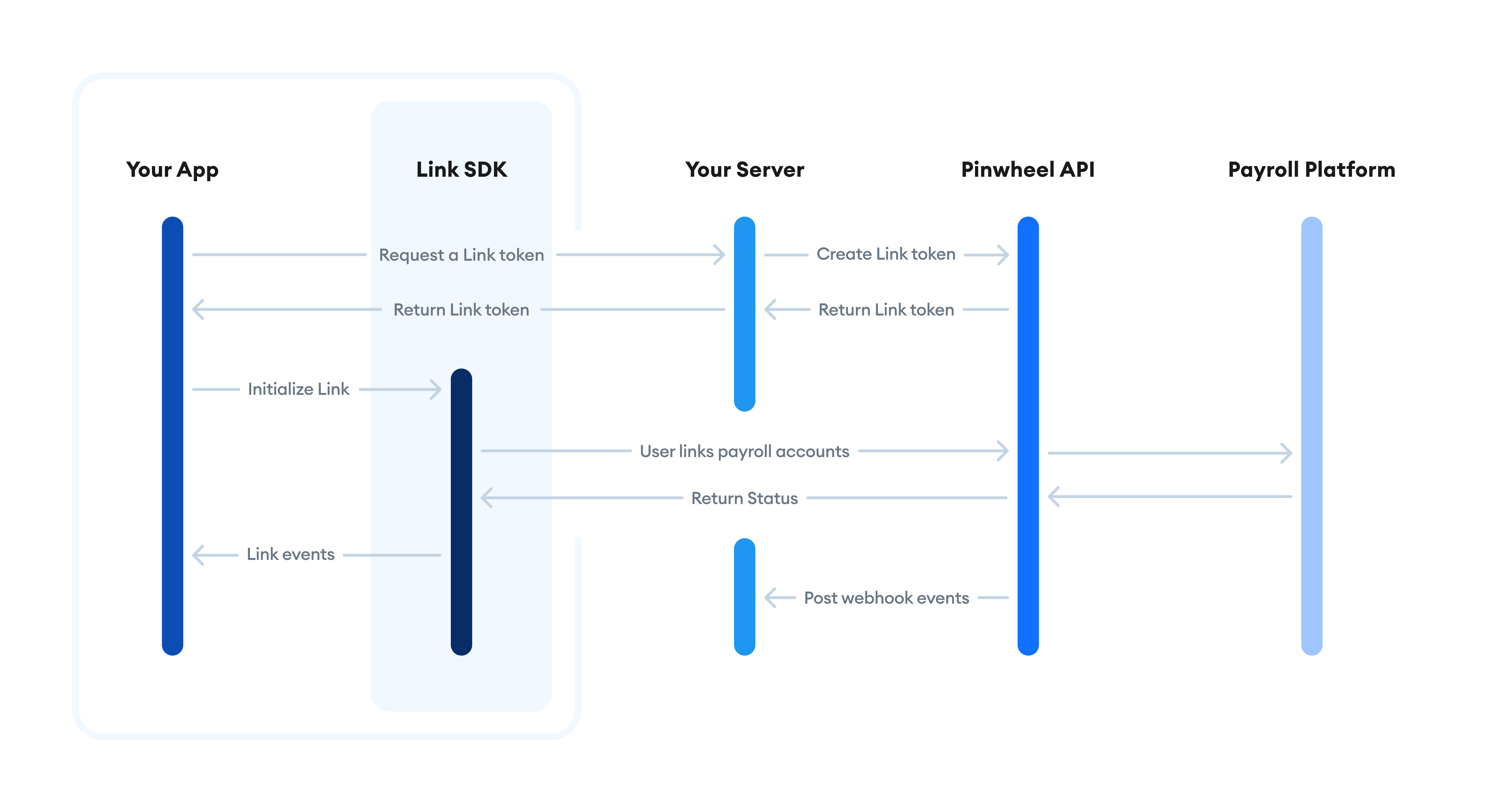
Prerequisites
Link SDK version 2.0
In order to enable Verification Reports, the Link SDK used in your application must be upgraded to version 2.0 or later. We recommend upgrading to the latest version for maximum conversion.
API and Webhook Version v2023-11-22
To use the latest functionality of Verification Reports, you should upgrade to v2023-11-22. Breaking changes for each API version upgrade are listed in our breaking change list. Functionality of older versions can be found by using the API version dropdown in the top left corner of this page.
Step 1: Subscribe to webhook events
Subscribe to Pinwheel Verify Webhook Events to receive instant notifications when up-to-date Verify data is available or Monitoring detects a change in a user's information.
verification_reports.refreshed
: Notifies you when a verification report is refreshed.
Register a webhook endpoint using Pinwheel's Create Webhook, as the following example illustrates.
POST /v1/webhooks
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"url": "https://your-domain.com/webhook_endpoint",
"status": "ACTIVE",
"enabled_events": [
"verification_reports.refreshed"
]
}
Step 2: Create a Link token
Create Link Token by sending the POST request to the link_tokens endpoint.
In the following request payload, you can see the fields that are required to create a link token for use with Pinwheel Verify:
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"required_jobs": ["employment", "income"]
}
org_name
: The name of your organization.required_jobs
: The jobs run to get employment and income data. At least one of these jobs is required.end_user_id
: The company's internal reference to the end user. See User Model for more information.language
- The language that the link modal uses.
The response to this call returns a link token you can use to launch the Link Modal in your app and a unique id
. Persist the id
in your database.
{
"data": {
"id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"expires": "2021-01-09T02:52:26+00:00",
"mode": "production",
"token": "eyJ0eXAiOiJKV1QiLCJhbGci...cyldX8fILelb6A0XKmdWsXZHMH4W1o",
}
}
Step 3: Initialize Link
Use the Link token to open the Link modal in your client application. In addition to passing in the token, you can optionally pass several callback handlers.
<!DOCTYPE html>
<html>
<head>
<script src="https://cdn.getpinwheel.com/pinwheel-v2.3.js"></script>
<script>
window.addEventListener("load", () => {
Pinwheel.open({
linkToken: "INSERT LINK TOKEN",
});
});
</script>
</head>
<body></body>
</html>
Calling Pinwheel.open()
renders the Link modal in full-screen mode.
Step 4: Respond to webhook events
The account.added
webhook event is published after a user logs in to their payroll account. Using the link_token_id
that you persisted earlier, associate the account with the user who logged in with Link.
{
"event": "account.added",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d",
"end_user_id": "my_user_12345",
"link_token_id": "97f420ff-5d0a-46ee-9cfc-6f17d5d31256",
"platform_id": "fce3eee0-285b-496f-9b36-30e976194736",
"created_at": "2021-01-12T02:36:01.287148+00:00",
"connected": true
}
}
Subsequent webhook events are published when data for a report are updated.
{
"event": "verification_reports.refreshed",
"event_id": "5a141122-4235-4fa1-bd76-0628573880b0",
"payload": {
"end_user_id": "my_user_12345",
"availability": {
"voe": {"status": "available", "updated_at": "2021-01-12T02:36:01.287148+00:00", "refreshed_at": "2021-01-12T02:36:01.287148+00:00"},
"voie": {"status": "available", "updated_at": "2021-01-12T02:36:01.287148+00:00", "refreshed_at": "2021-01-12T02:36:01.287148+00:00"}
}
}
}
Step 5: Retrieve data
To retrieve verification report data, you must use the end_user_id
from the webhook event you received and the endpoint associated with the data you're retrieving.
Here's an example of a verification of employment report:
Request
GET /v1/end_users/my_user_12345/verification_reports/voe
Host: api.getpinwheel.com
Content-Type: application/json
x-api-secret: YOUR-API-SECRET
Response
See the full Verification Reports API reference here.
{
"data": {
"document": {
"download_url": "download_url",
"download_url_expiration": "2024-01-06T17:47:52.782334+00:00",
"id": "15bbb566-fb4e-4835-9967-b308c164269e"
},
"employee": {
"address": {
"city": "Washington",
"country": "US",
"line1": "1234 Home Road",
"line2": null,
"postal_code": "20017",
"raw": "1234 Home Road, Washington, DC, 20017, USA",
"state": "DC"
},
"date_of_birth": "1987-02-01",
"last_four_ssn": "0090",
"name": {
"first_name": "Alicia",
"full_name": "Alicia Green",
"last_name": "Green",
"middle_name": ""
},
"phone_numbers": [
{
"type": "mobile",
"value": "+14155552671"
}
]
},
"employments": [
{
"employer_address": {
"city": "Washington",
"country": "US",
"line1": "1234 Main St",
"line2": "Suite 3",
"postal_code": "20036",
"raw": "1234 Main St, Suite 3, Washington, DC, 20036, USA",
"state": "DC"
},
"employer_name": "Acme Corporation",
"employment_duration_months": 23,
"employment_status": "employed",
"employment_type": "full time",
"most_recent_pay_date": "2021-01-05",
"start_date": "2020-06-01",
"termination_date": null,
"title": "Engineer"
}
],
"id": "aea8cc55-93fa-452d-8eac-b83c80b19a41",
"refreshed_at": "2021-01-06T15:59:13.530178+00:00",
"report_type": "voe",
"updated_at": "2021-01-06T15:59:13.530178+00:00"
}
}
Step 6 (Optional): Perform an On Demand Update
When a user's account loses connection or you need the latest information for a user, asking the user to log in multiple times introduces friction. Pinwheel has added the ability to reaccess the payroll account without needing the user to re-enter credentials.
Enabling this functionality is similar to creating standard Link tokens. To create the Link token, pass in the account_id
parameter from a prior account.added webhook event or from the login
client side event in Link. The account_id
can be preserved and passed in whenever you have it for a user.
POST /v1/link_tokens
Host: api.getpinwheel.com
Content-Type: application/json
X-API-SECRET: YOUR-API-SECRET
{
"org_name": "YOUR APP NAME",
"end_user_id": "my_user_12345",
"required_jobs": ["employment", "income"],
"account_id": "03bbc20e-bc39-464a-b4dc-4b63ffb7213d"
}
It is not necessary to pass end_user_id
when creating Link tokens for on-demand updates, as the information persists from the initial connection.
Once you've created the Link token, follow Step 3 to initialize the Link. When the user is presented with the Pinwheel Link flow, they are directed to the payroll platform associated with their account, bypassing login if possible.
Updated 9 months ago